Some applications may require to provide users with quick editing capabilities. While Inline Edit does just that entering inline edit mode for multiple records can be painful. It would be much easier is some or all fields appear as edit controls when page is loaded.
While PHPRunner/ASPRunner.NET/ASPRunnerPro do not have such functionality built-in it's fairly easy to implement it in your project. In this sample project we'll show how to make fields ProductName, UnitPrice and Discontinued editable automatically. For now we only support text boxes and check boxes. Data is saved automatically once you leave the text box or check off check box. To see that data is actually saved in the database simply reload the page.
You can also see how server-side validation works. Enter Unit Price that is less than $20 and move to the next field to see it in action. Record won't be saved until you enter $20 or more price value.
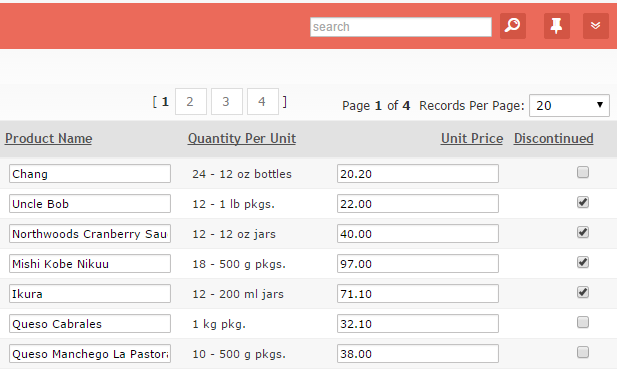
1. 'View as' Custom
For each field that you want to edit on the List page set 'View as' type 'Custom'. The code below is for PHPRunner only now but we'll update it with ASP/C# code shortly.
Check box field code
- $field="Discontinued";
- $keyField="ProductID";
- $value = "<input data-fieldname='".$field."' type=checkbox data-editid='".
- $data[$keyField]."' class='chAutoUpdate' ";
- if ($data[$field])
- $value.="checked ";
- $value.=">";
Text box field code
- $field="ProductName";
- $keyField="ProductID";
- $value = "<input data-fieldname='".$field."' data-editid='".$data[$keyField]."'
- type=text class='txtAutoUpdate' value=\"".runner_htmlspecialchars($data[$field])."\">";
Obvious changes are field name and key column name. You can also change field styling, width etc.
2. List page Javascript OnLoad code
- var elem;
- $(document).ready(function() {
- $('.chAutoUpdate').change(function() {
- var id = $(this).attr("data-editid");
- var field=$(this).attr("data-fieldname");
- var val="";
- elem = $(this);
- if($(this).is(":checked")) {
- val="on";
- }
- var data = {
- id: 1,
- editType: "inline",
- a: "edited",
- editid1: id
- };
- data["value_"+field+"_1"]=val;
- data["type_"+field+"_1"]="checkbox";
- // save data
- $.ajax({
- type: "POST",
- url: "products_edit.php?submit=1",
- data: data
- }).done( function(jsondata) {
- var decoded = $('<div/>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- if (response["success"]==false) {
- $("<div class=rnr-error/>").insertAfter(elem).html(response["message"]);
- }
- });
- });
- $('.txtAutoUpdate').change(function() {
- var id = $(this).attr("data-editid");
- var val=$(this).val();
- var field=$(this).attr("data-fieldname");
- elem = $(this);
- var data = {
- id: 1,
- editType: "inline",
- a: "edited",
- editid1: id
- };
- data["value_"+field+"_1"]=val;
- // clear error message if any
- if ($(this).next().attr('class')=="rnr-error")
- $(this).next().remove();
- // save data
- $.ajax({
- type: "POST",
- url: "products_edit.php?submit=1",
- data: data
- }).done( function(jsondata) {
- var decoded = $('<div/>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- if (response["success"]==false) {
- $("<div class=rnr-error/>").insertAfter(elem).html(response["message"]);
- }
- });
- });
- });
Changes in the code above: replace products_edit.php with the name of your edit page.
3. Validation part
This part is optional but we post it to make example complete. This code can be pasted to BeforeEdit event and we make sure that price is $20 or greater.
- if (array_key_exists("UnitPrice", $values) ) {
- if ($values["UnitPrice"]<20.0) {
- $message="Price should be greater than 20.0";
- return false;
- }
- }
- return true;