Similar to Excel-like grid discussed in previous article this technique helps you to achieve similar goals. Instead of bringing up the whole edit page you can click that single field you need to edit, change its value and see your changes posted to the database automatically.
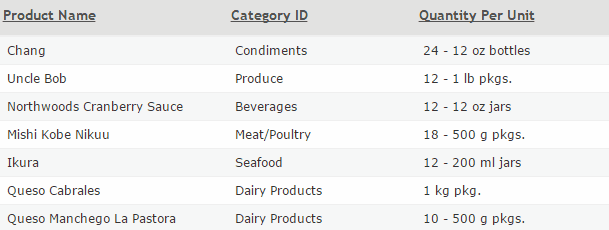
Click any of fields that are enabled for inline editing. Text will turn into edit control, the way it were setup in PHPRunner, ASPRunnerPro or ASPRunner.NET. To exit editing hit Esc button on your keyboard or click anywhere outside of edit control. Changes will be saved automatically.
To make this happen select one or more fields to be editable inline and add the following code to List page: Javascript OnLoad event.
Code
PHPRunner
- $(document).ready(function() {
- var $elem;
- var key;
- var field;
- var val;
- function cancelEditing() {
- if ($grid.data("editing")) {
- $elem.html(val);
- $grid.data("editing", false);
- $elem.closest("td").css("padding","5px 8px");
- }
- };
- $(document).keyup(function(e) {
- if (e.keyCode == 27) {
- cancelEditing();
- }
- });
- $("span[id^=edit]").attr('title', 'Click to edit');
- $grid=$(document.body);
- $grid.on("click", function(e) {
- // click during editing outside of edited element
- if ($grid.data("editing") &&
- !$(e.target).is(":focus") ) {
- cancelEditing();
- return;
- }
- // click on one of editable elements?
- if( $grid.data("editing") ||
- !$(e.target).attr("id") ||
- !$grid.data("editing") && $(e.target).attr("id").substring(0, 4) != "edit"
- ) {
- return;
- }
- $elem = $(e.target);
- // enter editing mode
- val = $elem.html();
- $grid.data("editing", true);
- var id = $elem.parent().attr("data-record-id");
- var res=$elem.attr("id").match(/[^_]+$/);
- field = res[0];
- var gridrows = JSON.parse(JSON.stringify(pageObj.controlsMap.gridRows));
- for (var i = 0; i < gridrows.length; i++) {
- if (gridrows[i].id==id) {
- key=gridrows[i].keys[0];
- break;
- }
- }
- // prepare request
- var data = {
- id: id,
- editType: "inline",
- editid1: key,
- isNeedSettings: true
- };
- // get edit controls from the server
- $.ajax({
- type: "POST",
- url: "products_edit.php",
- data: data
- }).done( function(jsondata) {
- var decoded = $('
- <div>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- // clear error message if any
- if ($elem.next().attr('class')=="rnr-error")
- $elem.next().remove();
- // cmake control as wide as enclosing table cell
- width = $elem.closest("td").width();
- $elem.html(response.html[field]);
- if (response.html[field].indexOf("checkbox")<0) {
- $elem.find("input,select").width(width-5).focus();
- $elem.closest("td").css("padding","1px 1px");
- }
- });
- });
- $grid.data("editing", false);
- // save function
- $(document.body).on('change','input[id^=value],select[id^=value]',function() {
- var data = {
- id: 1,
- editType: "inline",
- a: "edited",
- editid1: key
- };
- var type = $(this).attr('type');
- if (type)
- type=type.toLowerCase();
- else
- type="text";
- // regular field or check box
- if (type!="checkbox") {
- data["value_"+field+"_1"]=$(this).val();
- } else {
- if($(this).is(":checked")) {
- val="on";
- }
- data["value_"+field+"_1"]=val;
- data["type_"+field+"_1"]="checkbox";
- }
- // clear error message if any
- if ($(this).next().attr('class')=="rnr-error")
- $(this).next().remove();
- // save data
- $.ajax({
- type: "POST",
- url: "products_edit.php?submit=1",
- data: data
- }).done( function(jsondata) {
- var decoded = $('
- <div>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- if (response["success"]==false) {
- $("
- <div class="rnr-error/">").insertAfter($elem).html(response["message"]);
- }
- else {
- $elem.html(response["vals"][field]);
- $grid.data("editing", false);
- $elem.closest("td").css("padding","5px 8px");
- }
- });
- });
- });
- </div></div></div>
You will have to modify the Edit page URL accordingly to your project settings. Replace products_edit.phpwith corresponding name of your table i.e. tablename_edit.php.
ASPRunnerPro
Replace products_edit.php with corresponding name of your table i.e. tablename_edit.asp.
ASPRunner.NET
No changes are required. Code goes to List page: Javascript OnLoad event.
- $(document).ready(function() {
- var $elem;
- var key;
- var field;
- var val;
- function cancelEditing() {
- if ($grid.data("editing")) {
- $elem.html(val);
- $grid.data("editing", false);
- $elem.closest("td").css("padding","5px 8px");
- }
- };
- $(document).keyup(function(e) {
- if (e.keyCode == 27) {
- cancelEditing();
- }
- });
- $("span[id^=edit]").attr('title', 'Click to edit');
- $grid=$(document.body);
- $grid.on("click", function(e) {
- // click during editing outside of edited element
- if ($grid.data("editing") &&
- !$(e.target).is(":focus") ) {
- cancelEditing();
- return;
- }
- // click on one of editable elements?
- if( $grid.data("editing") ||
- !$(e.target).attr("id") ||
- !$grid.data("editing") && $(e.target).attr("id").substring(0, 4) != "edit"
- ) {
- return;
- }
- $elem = $(e.target);
- // enter editing mode
- val = $elem.html();
- $grid.data("editing", true);
- var id = $elem.parent().attr("data-record-id");
- var res=$elem.attr("id").match(/[^_]+$/);
- field = res[0];
- var gridrows = JSON.parse(JSON.stringify(pageObj.controlsMap.gridRows));
- for (var i = 0; i < gridrows.length; i++) {
- if (gridrows[i].id==id) {
- key=gridrows[i].keys[0];
- break;
- }
- }
- // prepare request
- var data = {
- id: id,
- editType: "inline",
- editid1: key,
- isNeedSettings: true
- };
- // get edit controls from the server
- $.ajax({
- type: "POST",
- url: "edit",
- data: data
- }).done( function(jsondata) {
- var decoded = $('
- <div>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- // clear error message if any
- if ($elem.next().attr('class')=="rnr-error")
- $elem.next().remove();
- // cmake control as wide as enclosing table cell
- width = $elem.closest("td").width();
- $elem.html(response.html[field]);
- if (response.html[field].indexOf("checkbox")<0) {
- $elem.find("input,select").width(width-5).focus();
- $elem.closest("td").css("padding","1px 1px");
- }
- });
- });
- $grid.data("editing", false);
- // save function
- $(document.body).on('change','input[id^=value],select[id^=value]',function() {
- var data = {
- id: 1,
- editType: "inline",
- a: "edited",
- editid1: key
- };
- var type = $(this).attr('type');
- if (type)
- type=type.toLowerCase();
- else
- type="text";
- // regular field or check box
- if (type!="checkbox") {
- data["value_"+field+"_1"]=$(this).val();
- } else {
- if($(this).is(":checked")) {
- val="on";
- }
- data["value_"+field+"_1"]=val;
- data["type_"+field+"_1"]="checkbox";
- }
- // clear error message if any
- if ($(this).next().attr('class')=="rnr-error")
- $(this).next().remove();
- // save data
- $.ajax({
- type: "POST",
- url: "edit?submit=1",
- data: data
- }).done( function(jsondata) {
- var decoded = $('
- <div>').html(jsondata).text();
- response = jQuery.parseJSON( decoded );
- if (response["success"]==false) {
- $("
- <div class="rnr-error/">").insertAfter($elem).html(response["message"]);
- }
- else {
- $elem.html(response["vals"][field]);
- $grid.data("editing", false);
- $elem.closest("td").css("padding","5px 8px");
- }
- });
- });
- });</div></div></div>